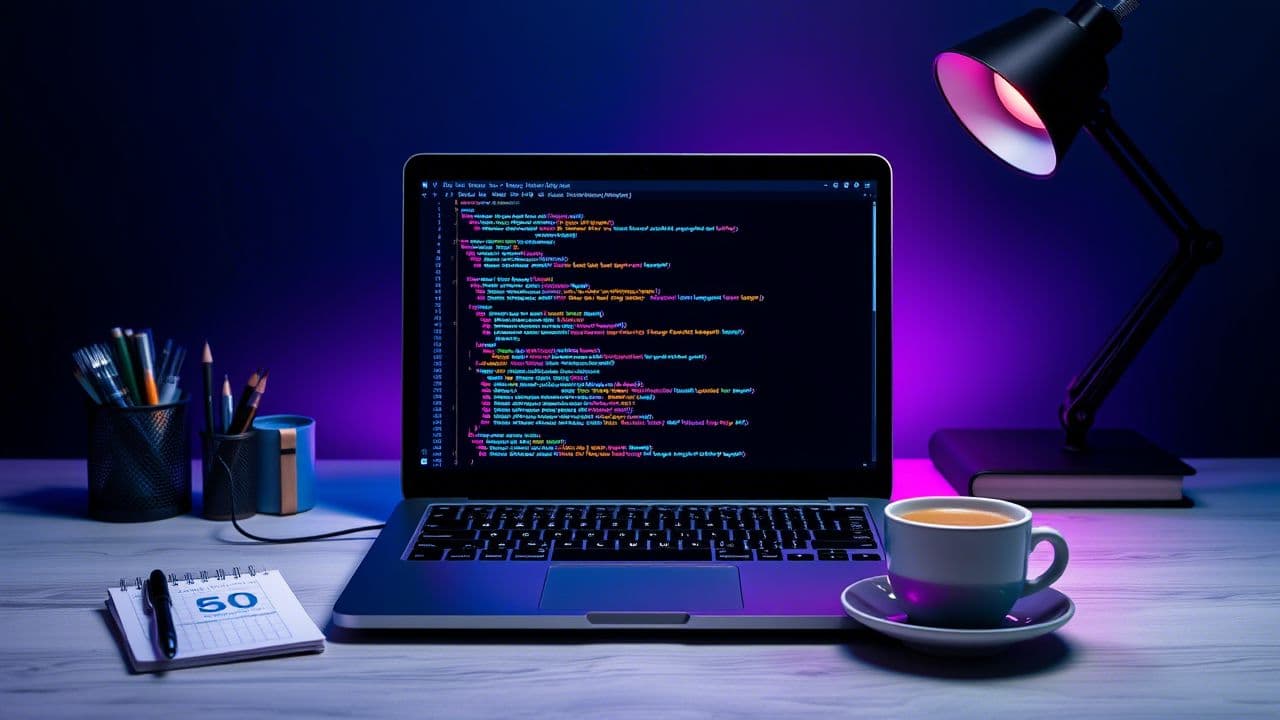
DA
dev Await
February 2025
Understanding React Keys: A Practical Guide
Introduction
In React, keys are special attributes that help React identify which items in a list have changed, been added, or removed. Think of keys as unique IDs for React elements, similar to how each product in a store has a unique barcode. Keys are essential when rendering lists of elements to help React update the DOM efficiently.
Where to Use Keys? 🎯
In Lists (map)
const fruits = ['Apple', 'Banana', 'Orange']; return ( <ul> {fruits.map((fruit, index) => ( <li key={index}>{fruit}</li> ))} </ul> );
Array of Objects
const users = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' } ]; return ( <div> {users.map(user => ( <div key={user.id}>{user.name}</div> ))} </div> );
Best Practices 💡
Use Unique IDs
// Good <div key={user.id}>{user.name}</div> // Bad <div key={Math.random()}>{user.name}</div>
Avoid Index as Keys for Dynamic Lists
// Not recommended for lists that can change {items.map((item, index) => ( <li key={index}>{item.name}</li> ))} // Better approach {items.map(item => ( <li key={item.id}>{item.name}</li> ))}
Why Keys Matter? 🤔
Helps React identify changes in lists
Improves performance
Maintains component state correctly
Prevents rendering bugs
That's the essential information about React keys! Remember, always use unique and stable values for keys when rendering lists. Happy coding! 🚀