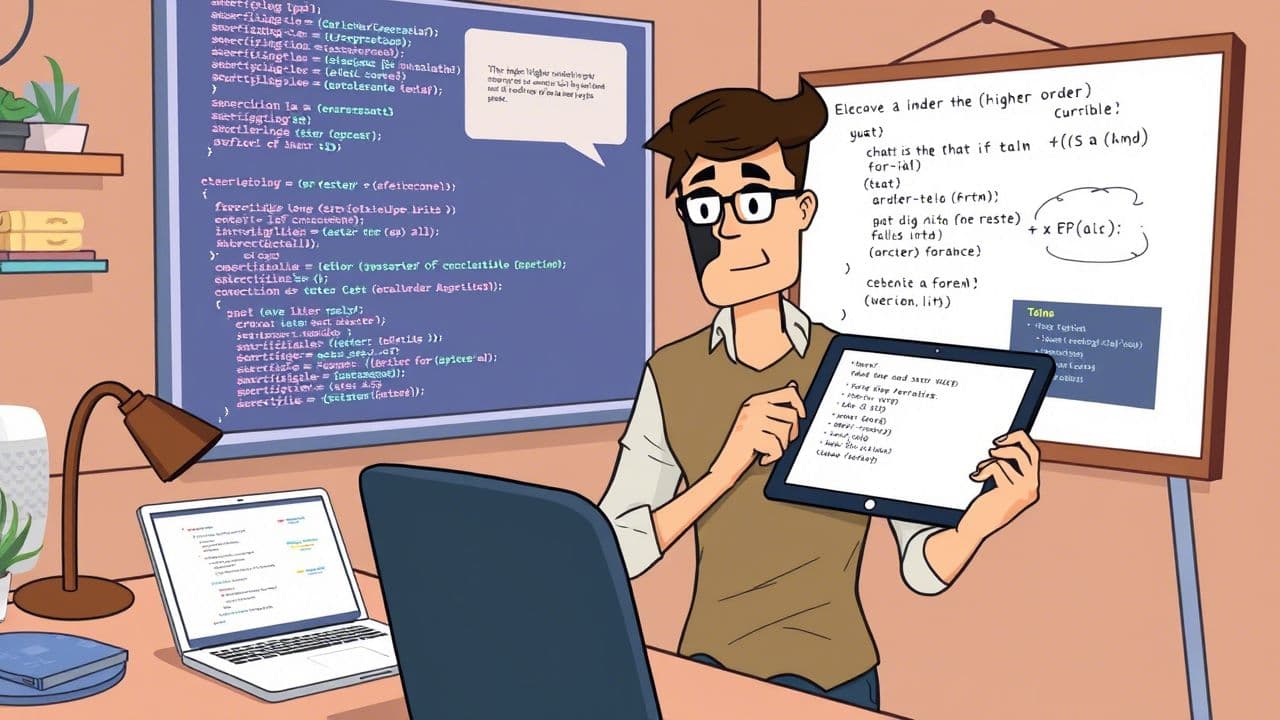
DA
dev Await
February 2025
Understanding Higher Order Functions
What is a Higher Order Function? 🤔
A higher-order function is a function that either:
Takes one or more functions as arguments
Returns a function
Or both
Simple Examples 💡
Function as an Argument
// A simple HOF that takes a function as an argument const calculate = (operation, a, b) => { return operation(a, b); }; // Functions to pass as arguments const add = (x, y) => x + y; const multiply = (x, y) => x * y; // Using the HOF console.log(calculate(add, 5, 3)); // Output: 8 console.log(calculate(multiply, 5, 3)); // Output: 15
Returning a Function
// HOF that returns a function const greet = (greeting) => { return (name) => { return `${greeting}, ${name}!`; }; }; const sayHello = greet('Hello'); const sayHi = greet('Hi'); console.log(sayHello('John')); // Output: Hello, John! console.log(sayHi('Jane')); // Output: Hi, Jane!
Common Built-in HOFs 🛠️
Array.map()
const numbers = [1, 2, 3, 4]; const doubled = numbers.map(num => num * 2); // Result: [2, 4, 6, 8]
Array.filter()
const numbers = [1, 2, 3, 4, 5, 6]; const evenNumbers = numbers.filter(num => num % 2 === 0); // Result: [2, 4, 6]
Array.reduce()
const numbers = [1, 2, 3, 4]; const sum = numbers.reduce((acc, curr) => acc + curr, 0); // Result: 10
Common Use Cases 🎯
Data transformation
Event handling
Component composition in React
Function composition
Middleware functions
Benefits 📈
Better code reusability
More modular code
Enhanced code flexibility
Cleaner code structure
Better separation of concerns
Tips 💡
Keep functions pure when possible
Use meaningful names for parameters
Don't over-complicate function composition
Handle edge cases appropriately
Document your HOFs well