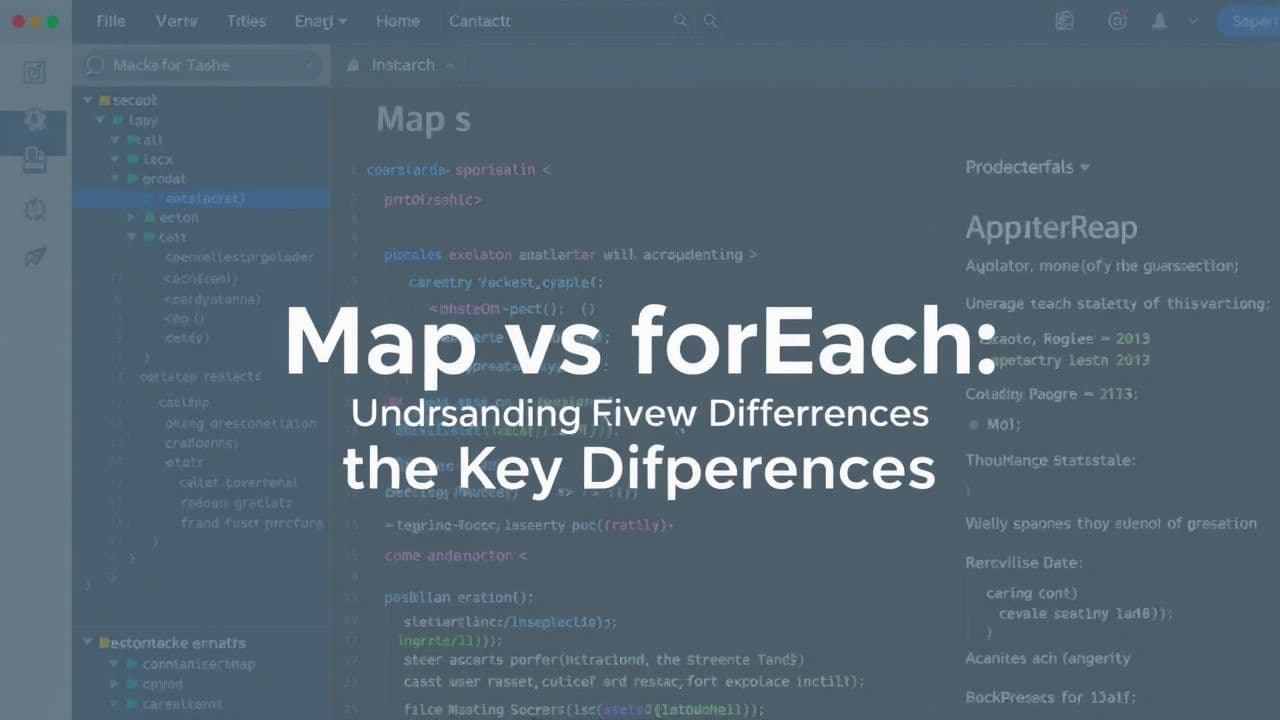
dev Await
February 2025
Map vs forEach: Understanding the Key Differences
Introduction
map() and forEach() are two essential array methods in JavaScript that help you work with arrays, but they serve different purposes and have distinct behaviors. While both methods iterate over array elements, map() is designed for transforming data and creating new arrays, whereas forEach() is meant for executing actions on array elements without creating a new array. Understanding these differences is crucial for writing efficient and maintainable code, especially in React applications where data transformation is common.
Main Differences 🎯
Return Value
//map() returns a new array const numbers = [1, 2, 3]; const doubled = numbers.map(num => num * 2); console.log(doubled); // [2, 4, 6] // forEach() returns undefined const numbers2 = [1, 2, 3]; const result = numbers2.forEach(num => num * 2); console.log(result); // undefined
Chaining
// map() can be chained const numbers = [1, 2, 3]; const result = numbers .map(num => num * 2) .filter(num => num > 4); // forEach() cannot be chained numbers.forEach(num => num * 2).filter(); // Error!
Array Modification
// map() creates new array const numbers = [1, 2, 3]; const newArray = numbers.map(num => num * 2); console.log(numbers); // [1, 2, 3] console.log(newArray); // [2, 4, 6] // forEach() modifies existing array const numbers2 = [1, 2, 3]; numbers2.forEach((num, index, arr) => { arr[index] = num * 2; }); console.log(numbers2); // [2, 4, 6]
When to Use Each 🤔
Use map() when:
You want to create a new array
You need to transform data
You plan to chain operations
You need to use the returned array
// Good use of map() const users = [ { id: 1, name: 'John' }, { id: 2, name: 'Jane' } ]; const userNames = users.map(user => user.name);
Use forEach() when:
You just want to iterate over elements
You don't need a new array
You're performing side effects
You're modifying the original array
// Good use of forEach() const numbers = [1, 2, 3]; let sum = 0; numbers.forEach(num => { sum += num; });
Quick Example in React
// Using map() (Good)
const UserList = ({ users }) => {
return (
<ul>
{users.map(user => (
<li key={user.id}>{user.name}</li>
))}
</ul>
);
};
// Using forEach() (Bad Practice in React)
const UserList = ({ users }) => {
const listItems = [];
users.forEach(user => {
listItems.push(<li key={user.id}>{user.name}</li>);
});
return <ul>{listItems}</ul>;
};
Tips 💡
Use map() for transforming data into new arrays
Use forEach() for side effects (like logging)
map() is more functional programming friendly
forEach() is better for imperative programming
In React, prefer map() for rendering lists