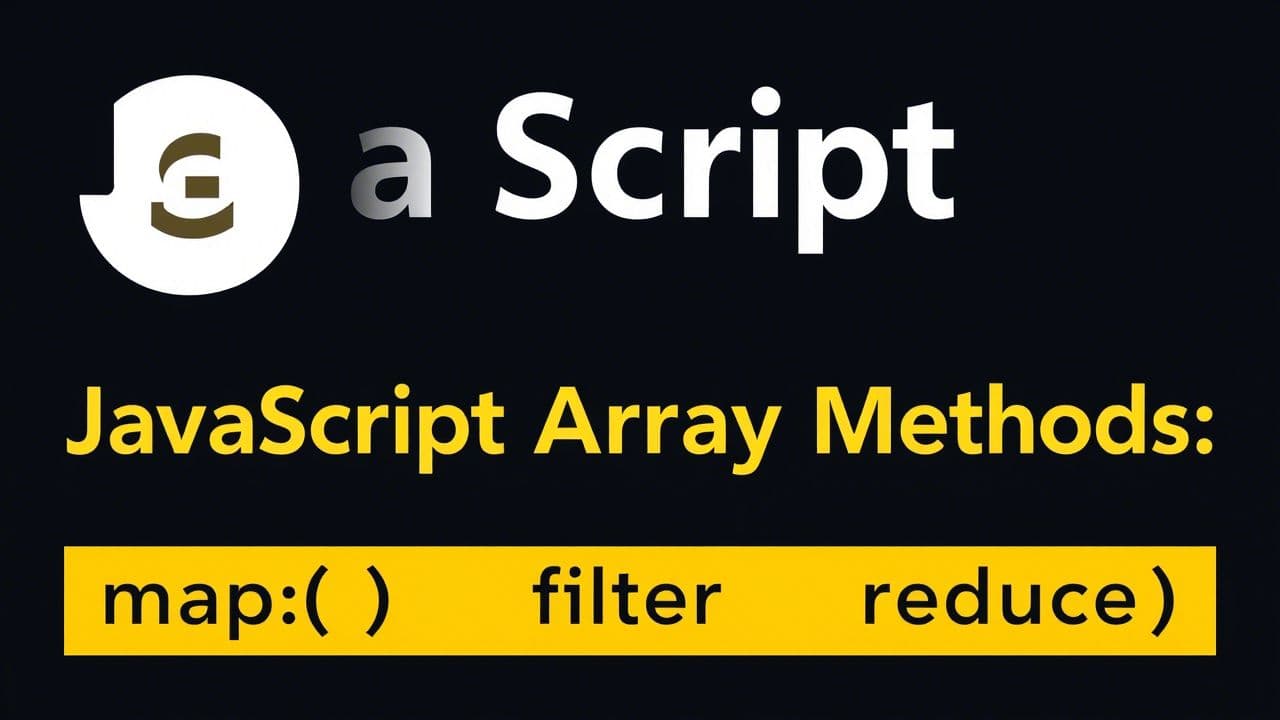
dev Await
February 2025
🔄 JavaScript Array Methods: map(), filter(), reduce()
JavaScript array methods (map, filter, and reduce) are powerful built-in functions that help you manipulate and transform arrays. These methods are essential tools in modern JavaScript and React development, making it easier to handle data transformations, filtering, and calculations. Instead of using traditional for loops, these methods provide a more readable and functional approach to array operations. You'll commonly use map() when you need to transform each item in an array (like displaying a list of users), filter() when you need to selectively show items based on conditions (like showing only active users), and reduce() when you need to combine array elements into a single value (like calculating a shopping cart total).
map() Method 🔄
Transforms each element in an array to create a new array.
// Basic Example const numbers = [1, 2, 3, 4]; const doubled = numbers.map(num => num * 2); console.log(doubled); // [2, 4, 6, 8] // React Example const names = ['John', 'Jane', 'Bob']; return ( <ul> {names.map((name, index) => ( <li key={index}>{name}</li> ))} </ul> );
filter() Method 🔍
Creates new array with elements that pass a condition.
// Basic Example const numbers = [1, 2, 3, 4, 5, 6]; const evenNumbers = numbers.filter(num => num % 2 === 0); console.log(evenNumbers); // [2, 4, 6] // React Example const users = [ { id: 1, name: 'John', age: 25 }, { id: 2, name: 'Jane', age: 17 }, { id: 3, name: 'Bob', age: 30 } ]; const adults = users.filter(user => user.age >= 18);
reduce() Method 📊
Reduces array to a single value.
// Basic Example const numbers = [1, 2, 3, 4]; const sum = numbers.reduce((total, num) => total + num, 0); console.log(sum); // 10 // Shopping Cart Example const cart = [ { item: 'Book', price: 10 }, { item: 'Pen', price: 2 }, { item: 'Notebook', price: 5 } ]; const total = cart.reduce((sum, item) => sum + item.price, 0); console.log(total); // 17
Common Use Cases 🎯
map()
Rendering lists of components
Transforming data for display
Creating new arrays with modified data
filter()
Filtering items based on search
Showing completed/incomplete todos
Filtering based on categories
reduce()
Calculating totals
Grouping data
Converting array to object